Handling Case Objects That Use Inheritance in Scripts
From within a script, use the classNameRef type-casting method for the appropriate case access class to return a case reference of the specialized class type from a case reference of the general class type.
Method | Description |
---|---|
classNameRef(caseRef) | Returns a case reference of the className type for the specified caseRef. |
Case classes can use generalization relationships to provide specialization and inheritance. For example, in the following case data model the Employee and Customer classes are specializations of the (general) Person class. Note that they both inherit a searchable attribute (name) and their case identifier (Id) from the parent Person class.
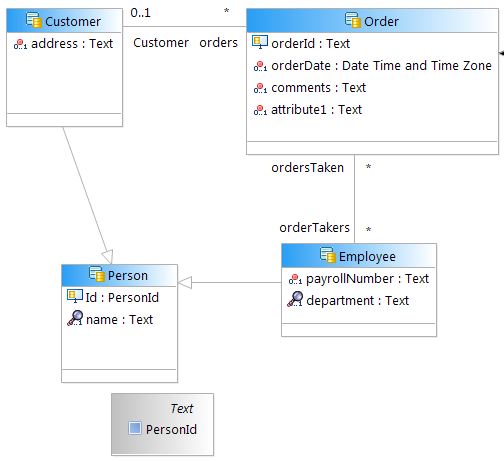
In a script, if you use the findAll() or find() methods to retrieve lists of case references for a general class type, each case reference in the list could be a reference to an object that belongs to either the general class itself, or to any specialized class derived from that class. In the example above, a list of Person case references could actually contain a mixture of Person, Customer and Employee references.
When processing the list, you may want to do different things depending on what type of class you are processing. To do this:
- Use the className or simpleClassName attributes to determine which class type a particular case reference points to
- Use the classNameRef type-casting method for the appropriate case access class to return a case reference of the specialized class type from a case reference of the general class type.
- Use the type-specific methods provided by the appropriate case access class to perform the desired processing.
Example
This example shows how to take a list of PersonRef case references, determine whether each is an Employee or a Customer and provide appropriate processing for each.
var personRefList = cac_com_example_ordermodel_Person.findAll(); // Process the list of PersonRefs (each of which which can be either be a CustomerRef or an EmployeeRef) for (var ix = 0; ix < personRefList.size(); ix++) { var personRef = personRefList.get(ix); // Use simpleClassName to determine whether this personRef is a CustomerRef or EmployeeRef if (personRef.simpleClassName == "Customer") { var customerRef = cac_com_example_ordermodel_Customer.customerRef(personRef); // Process customerRef specifics... } else if (personRef.simpleClassName == "Employee") { var employeeRef = cac_com_example_ordermodel_Employee.employeeRef(personRef); // Process employeeRef specifics... } }