Notifying a Process That a Case Object It Uses Has Been Modified
You can add a case data signal event handler to a process so that it can subscribe to a particular case object that the process uses. The process will then be notified if that case object is modified, and can take appropriate action to respond to the change.
- initialized when the case reference field is first assigned.
- re-initialized if the case reference field is changed to reference a different case object.
- uninitialized if the case reference field is set to null.
While the case data signal is initialized, the event is triggered whenever the referenced case object is either updated, linked to or unlinked from other case objects, or (in some circumstances) deleted.
Prerequisites
The process must already contain a data field of type Case Class Reference that identifies the case object to which the case data signal event handler will subscribe.
Procedure
Example
The following process fragment is part of a business process that handles a customer enquiry.
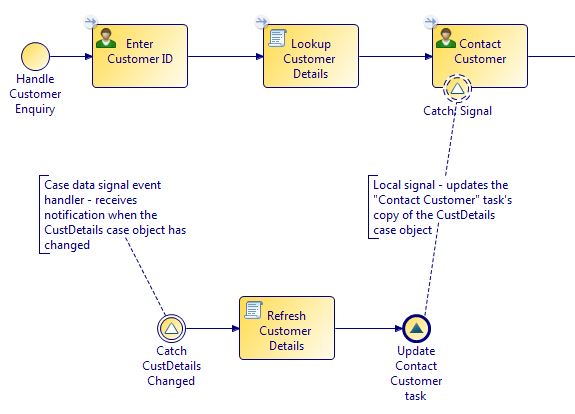
The main Handle Customer Enquiry process flow works like this:
- A customer support representative (CSR) dealing with the enquiry enters the customer's ID, which is then used in the
Lookup Customer Details script task to retrieve a case object containing the customer's details:
// Get the case reference to the CustDetails class that matches the identifier provided in the Customer ID (custID) field. custRef = cac_com_example_caseclass_CustomerDetails.findByCustID(CustID); // Read the case reference to get the corresponding CustomerDetails case data object. CustDetails = custRef.readCustomerDetails();
- The customer's details are displayed to the CSR in the Contact Customer task, which is offered to the CSR pool of workers.
To cater for the possibility that a customer's details could change in between the time that a Contact Customer work item is scheduled and a CSR opening and completing that work item, a case data signal event handler is implemented:
- The Catch CustDetails Changed event subscribes to the custRef case reference. It is triggered whenever the referenced CustDetails case object changes.
- The Refresh Customer Details script task interrogates the received event to find out if it was caused by an update. If it was, it re-reads the customer's details from the referenced case object and updates the local
CustDetails data field.
if // Check if the change that triggered the event was an update to the case object (CaseSignalAttributes.isCaseUpdate()) { CustDetails = custRef.readCustomerDetails(); } else { // Optionally perform other activities to handle the event if the case object has been // linked to or unlinked from other case objects, or deleted }
- The Update Contact Customer task throw signal event sends the updated CustDetails data field to its corresponding Catch Signal event, which is configured to update that data in the Contact Customer work item.
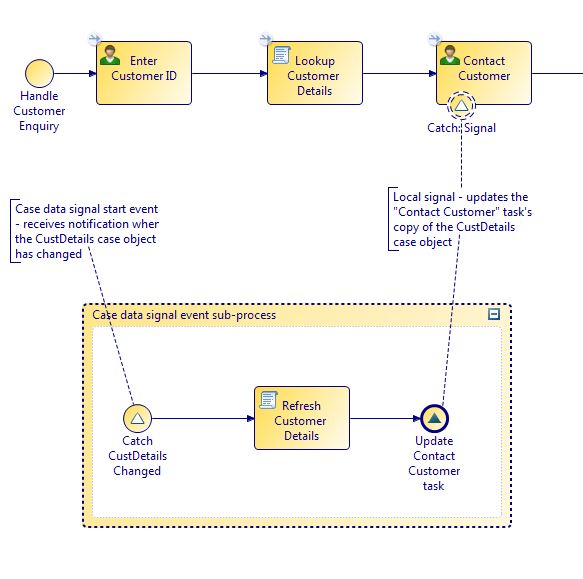