Multiple Instances of a BOM Class in a Process Data Field
If a process data field is flagged as being an Array Field when the process data field is created (or its properties are changed later), then instead of referring to a single Business Object, the process data field will refer to multiple instances of the Business Object. This is done through a List object, which can contain multiple Business Objects.
Let us consider a process data field that holds a List of Customer objects called custList. The properties sheet for custList has Array selected and is of type Customer:
Array fields with a multiplicity greater than 1 are implemented using the List object. The List objects do not need to be created. They are created by default as empty Lists. If you want to associate a particular Customer with the custList variable, you can assign the cust field with a single instance field. This is shown below.
cust = com_example_scriptingguide_Factory.createCustomer(); cust.name = "Fred Blogs"; cust.custNumber = "C123456"; |
Another way is to add the new customer to the custList. We can add multiple customers to a list as well, as shown below:
// Using cust variable created above (Fred Blogs / C123456) is // added to the List custList: custList.add(cust); // Now add a second customer to the list (John Smith): cust2 = com_example_scriptingguide_Factory.createCustomer(); cust2.name = "John Smith"; cust2.custNumber = "C123457"; custList.add(cust2); |
This can be pictured as follows:
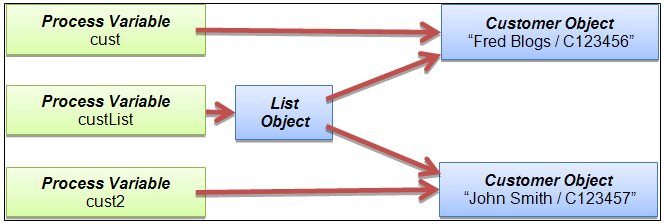
WARNING: If, after you used the script above, you then used the following script to add a third customer to the list, this would go wrong on two accounts.
First, a new Customer instance has not been created for Clint Hill, so the first two lines above modify the John Smith Customer instance to refer to Clint Hill. Then when the add() method is called for the third time, it will attempt to add a second reference to the same Customer instance. However, this add will fail because the List type used does not allow the same object to be included more than once. So the list ends up containing the Fred Blogs and Clint Hill Customer instances but not John Smith:
Instead, a Customer instance must be created using the factory method for each Customer to be added to the list. If references to the individual customer cust are not required outside of the script then local script, variables can be used in place of the process variable cust. The example below shows two script local variables c1 and c2 being used to correctly add two Customers to a custList:
// Create first customer instance var c1 = com_example_scriptingguide_Factory.createCustomer(); c1.name = "Fred Blogs"; c1.custNumber = "C123456"; custList.add(c1); // Create second customer instance var c2 = com_example_scriptingguide_Factory.createCustomer(); c2.name = "John Smith"; c2.custNumber = "C567890"; custList.add(c2); |
It is not necessary to use different variable names for the two Customer instances, variable c could have been used throughout the script in place of c1 and c2, but then the word var would have to be removed from the line that contains the second call to the createCustomer():
var c = com_example_scriptingguide_Factory.createCustomer(); c.name = "Fred Blogs"; c.custNumber = "C123456"; custList.add(c); c = com_example_scriptingguide_Factory.createCustomer(); c.name = "John Smith"; c.custNumber = "C567890"; custList.add(c); |
Another way of creating the second and subsequent instances would be to make copies of the first. This can be useful if there are a lot of attributes with the same value, for example:
// Create first customer instance var c1 = com_example_scriptingguide_Factory.createCustomer(); c1.name = "Fred Blogs"; c1.custNumber = "C123456"; c1.isRetail = true; c1.dateAdded = DateTimeUtil.createDate(); custList.add(c1); // Create second customer instance by copying the first var c2 = ScriptUtil.copy(c1); c2.name = "John Smith"; c2.custNumber = "C567890"; custList.add(c2); |
We can use the same var to store the new customer once the first customer has been added to the list. We do not need to keep a reference to it any longer:
// Create first customer instance var cust = com_example_scriptingguide_Factory.createCustomer(); cust.name = "Fred Blogs"; cust.custNumber = "C123456"; cust.isRetail = true; cust.dateAdded = DateTimeUtil.createDate(); custList.add(cust); // Create second customer instance by copying the first cust = ScriptUtil.copy(cust); cust.name = "John Smith"; cust.custNumber = "C567890"; custList.add(cust); |