Interface SessionInteraction
- Input parameters are set when the work item is started, and will be available during the work item lifespan.
- Internal parameters register the internal state of the work item, they can be set and accessed at any time of its lifespan.
- Output parameters must be set when the work item is considered complete. They will then be used by the running workflow to update its data context.
The overall development process is as follows:
- For a user service to be integrated into the workflow, it must first
be declared in the document
module.xml
, in particular its input and output parameters. - Once this declaration has been made, the user service becomes available in the Workflow Models area where the user defines user tasks, so that the input parameters and output parameters be mapped with the workflow data context.
- For the development itself, any component having access to a user session
can obtain the underlying interaction by means of the method
Session.getInteraction
.
For more information, see Parameters declaration and availability in workflows and perspectives.
Interaction life cycle
The following diagram shows the states of an interaction:
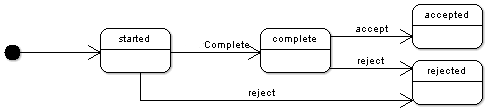
When a user starts a work item:
- a new
session
is created on the application server with an underlying interaction; - this interaction's
input parameters
are assigned values according to the mapping specified by the user task definition in the workflow model.
As long as the interaction is in the 'started' state, it means that the user has some work to perform.
When the interaction is considered to be complete the user service must invoke the method
complete
(this method can be invoked at the first HTTP request, if the interaction does not require any particular work).
Then, from the 'complete' state, the user can either
accept or reject it. It is possible to specify particular checks on
these actions by means of the method UserTask.checkBeforeWorkItemCompletion
.
Note: by default, 'Reject' is disabled (the button is not displayed); in the workflow model, it can be enabled in the user task definition.
Restoring an interrupted interaction
The session interaction is persistent, thus if the user closes the browser or logs out, or if the server is restarted, the last saved state can be used by a user service to correctly restore the same position when the user returns to the work item.
If a user service is composed of more than one simple step, it is recommended to use the appropriate methods to save the state at the end of each step and to retrieve this state in the first HTTP request if the work item is continued later. It is important for User services that are integrated with workflows to always use session interactions.
-
Nested Class Summary
Nested ClassesModifier and TypeInterfaceDescriptionstatic interface
Contains the report of a decision request (accept or reject).static interface
Gives information about the specification of the interaction. -
Method Summary
Modifier and TypeMethodDescriptionaccept()
Requests the interaction to be accepted and returns whether the operation has succeeded or not.void
complete
(InteractionHelper.ParametersMap outputParameters) Sets this interaction to the state 'complete'.Returns the comment of this interaction.Returns the initial input parameters of this interaction.Returns the internals parameters of this interaction.Returns the service key of this interaction.Returns the specification of the interaction given by the associated definition.Returns the work item key associated to the current session interaction.boolean
Returnstrue
if this interaction has been set to 'complete'.reject()
Requests the interaction to be rejected and returns whether the operation has succeeded.void
setComment
(String comment) Sets the comment of this interaction.void
setInternalParameters
(InteractionHelper.ParametersMap internalParameters) Sets the internal parameters of this interaction.
-
Method Details
-
getInputParameters
Returns the initial input parameters of this interaction.This method can be used by a user service started by a work item (for example, a trigger, an access rule, or on a specific service context) to read the input interaction properties.
- Returns:
- the input parameters map.
- Throws:
InteractionException
- See Also:
-
getComment
String getComment()Returns the comment of this interaction.The value of this comment is equal to the value entered by the user by clicking on the 'comment' button when the associated work item is open, or to the value updated by the method
setComment(String)
.- Since:
- 5.4.3
-
setComment
Sets the comment of this interaction. The call of this method will overwrite the existing comment if the user has already entered one.The new value of the comment will be displayed if the user clicks on the 'comment' button when the associated work item is open.
- Throws:
InteractionException
- if the interaction is not found.IllegalStateException
- if this method is called from a read-only context.- Since:
- 5.7.1
-
getServiceKey
Returns the service key of this interaction.This method can be used to test the invocation context of the interaction (creation, validation, editing, etc.) and perform specific operations according to the different contexts.
- Returns:
- the service key of this interaction, or
null
if the service is 'default'. If the user service of the interaction is an extension, it returns the service key extended (and not the service key of the extension) - Throws:
InteractionException
-
getSpecification
SessionInteraction.Specification getSpecification()Returns the specification of the interaction given by the associated definition.- Since:
- 5.8.0
-
getInternalParameters
Returns the internals parameters of this interaction. The map is not automatically initialized (null
by default). UsesetInternalParameters
to initialize it.This method can be used to read the internal interaction properties, when invoked in the context of the user service started by a workflow work item (for example, a trigger, an access rule, or on a specific service context).
- Returns:
- the internal parameters map.
- Throws:
InteractionException
-
setInternalParameters
void setInternalParameters(InteractionHelper.ParametersMap internalParameters) throws InteractionException Sets the internal parameters of this interaction.At the end of the work item, when the user accepts or rejects, the internal parameters which do not already exist in the output parameters are copied into the output parameters. Thus, internal parameters are available in the output of the interaction.
This method can be used to update the internal interaction properties, when invoked in the context of the user service started by a workflow work item (for example, in a trigger, in an access rule or on a specific service context).
- Parameters:
internalParameters
- specifies the internal parameters, used during the execution of the service.- Throws:
IllegalStateException
- if this method is called from a read-only context.InteractionException
-
complete
void complete(InteractionHelper.ParametersMap outputParameters) throws InteractionException, IllegalInteractionStateException Sets this interaction to the state 'complete'.In a specific user service, this method must be invoked so that the 'Accept' button is displayed.
If this method is called several times, the output parameters are updated incrementally (existing entries are updated and new entries are added).
This method can only be invoked for a session interaction opened in a work item user interface (managed by
and not by Java API
).- Parameters:
outputParameters
- specifies the result of the interaction; it must conform to the declaration of the service (properties with theoutput
attribute set totrue
).- Throws:
IllegalInteractionStateException
- if the interaction is already accepted or rejected (final states). In this case, the completion of the interaction is not possible anymore.InteractionException
- if the interaction is not found, or if an output parameter is not found in the associated service declaration.IllegalStateException
- if this method is called from a read-only context.UnsupportedOperationException
- if this method is called from a session interaction managed by Java API (not managed bySession.getInteraction(boolean)
).
-
isComplete
Returnstrue
if this interaction has been set to 'complete'.- Returns:
true
if the interaction has been set as complete, else returnsfalse
.- Throws:
InteractionException
- See Also:
-
accept
SessionInteraction.DecisionResult accept()Requests the interaction to be accepted and returns whether the operation has succeeded or not.This method can be invoked only for a session interaction in the 'complete' state and retrieved by
WorkflowEngine
(not accessed bySession.getInteraction(boolean)
).Before accepting the interaction, the following steps are performed:
- Checks if the comment is mandatory: if the comment is required but empty, an error message is reported in the result and the accept action is not done.
- Checks specific constraints of the user task. If constraints are not valid, error messages are reported in the result and the accept action is not done.
- Calls the workflow trigger. If the workflow trigger throws an exception, the exception is reported in the result and the accept action is not done.
- Returns:
- the result of the accept request.
- Throws:
UnsupportedOperationException
- if the session interaction has not been obtained byWorkflowEngine
.IllegalStateException
- if the interaction is not in the complete state.- Since:
- 5.7.1 fix D
- See Also:
-
reject
SessionInteraction.DecisionResult reject()Requests the interaction to be rejected and returns whether the operation has succeeded.This method can be invoked only for a session interaction retrieved by
Before rejecting the interaction, the following steps are performed:WorkflowEngine
(not accessed bySession.getInteraction(boolean)
).- Checks if the comment is mandatory: if the comment is required but empty, an error message is reported in the result and the reject action is not done.
- Checks specific constraints of the user task. If constraints are not valid, error messages are reported in the result and the reject action is not done.
- Calls the workflow trigger. If the workflow trigger throws an exception, the exception is reported in the result and the reject action is not done.
- Returns:
- the result of the reject request.
- Throws:
UnsupportedOperationException
- if the session interaction has not been obtained byWorkflowEngine
.IllegalStateException
- if the interaction is neither in the 'started' nor in the complete state, or the reject is disabled.- Since:
- 5.7.1 fix D
- See Also:
-
getWorkItemKey
WorkItemKey getWorkItemKey()Returns the work item key associated to the current session interaction.- Returns:
- the current work item key associated to the session interaction.
- Throws:
IllegalStateException
- if the interaction has no underlying work item.- Since:
- 6.0.1
-