Finding Case Objects by Example
From within a script, use the find method on the appropriate case access class to return a list of case references whose searchable atributes match the searchable attributes set in a local data object instance of the case class.
Method syntax | Description |
---|---|
find(localObject) | Returns a single list containing all matching case references. |
find(localObject,index,pageSize) | Returns a paginated list of matching case references. |
where:
- localObject is a local data object instance of the case class, containing one or more searchable attributes set to the values to be used to find matching case objects. If multiple searchable attributes are specified, the case objects must match all specified values.
- index is the (zero-based) number of the first record to return in a paginated list.
- pageSize is the number of records to return in each page of a paginated list. A value of -1 means return all records.
Note: These methods are not sophisticated enough to find case objects based on searchable attributes specified in global objects contained in a case data object. To do this, you must use the
findByCriteria method. See
Finding Case Objects by Criteria.
Example
This example uses the following case class:
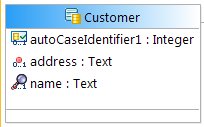
The script finds all the customers with a name of "Harris", and returns a list of references to them:
// Create a local data object instance of the Customer case class. var customer = com_example_ordermodel_Factory.createCustomer(); // Set the name field to the value you want to search on. 'name' must be a searchable attribute // on the Customer class. 'address' is not searchable and so cannot be used. customer.name = 'Harris'; // Get a list of all Customer case references that contain the name 'Harris'. var customerReferenceList = cac_com_example_ordermodel_Customer.find(customer); // You can now read the list of case references to obtain a list of corresponding Customer case data objects. var customerList = cac_com_example_ordermodel_Customer.read(customerReferenceList); // Then process matching customers. for (var ix = 0; ix < customerList.size(); ix++) { var customer = customerList.get(ix); // process customer objects… }
Copyright © Cloud Software Group, Inc. All rights reserved.